React’s been a powerhouse for building web apps over the last ten years.
We’ve all seen it evolve from those clunky class components to the elegance of hooks.
But React Server Components (RSCs)?
We don’t think anyone expected such a dramatic shift in how React worked.
So, what exactly are React Server Components? How do they work? And what do they do differently that React couldn’t already do?
To answer all these questions, we’ll quickly go over the fundamentals. If you’re in need of a refresher, have a quick look at this guide on how to learn React as a beginner.
In this post, we’ll walk you through why we needed React Server Components, how they work, and some of the major benefits of RSCs.
Let’s get started!
What Are React Server Components?
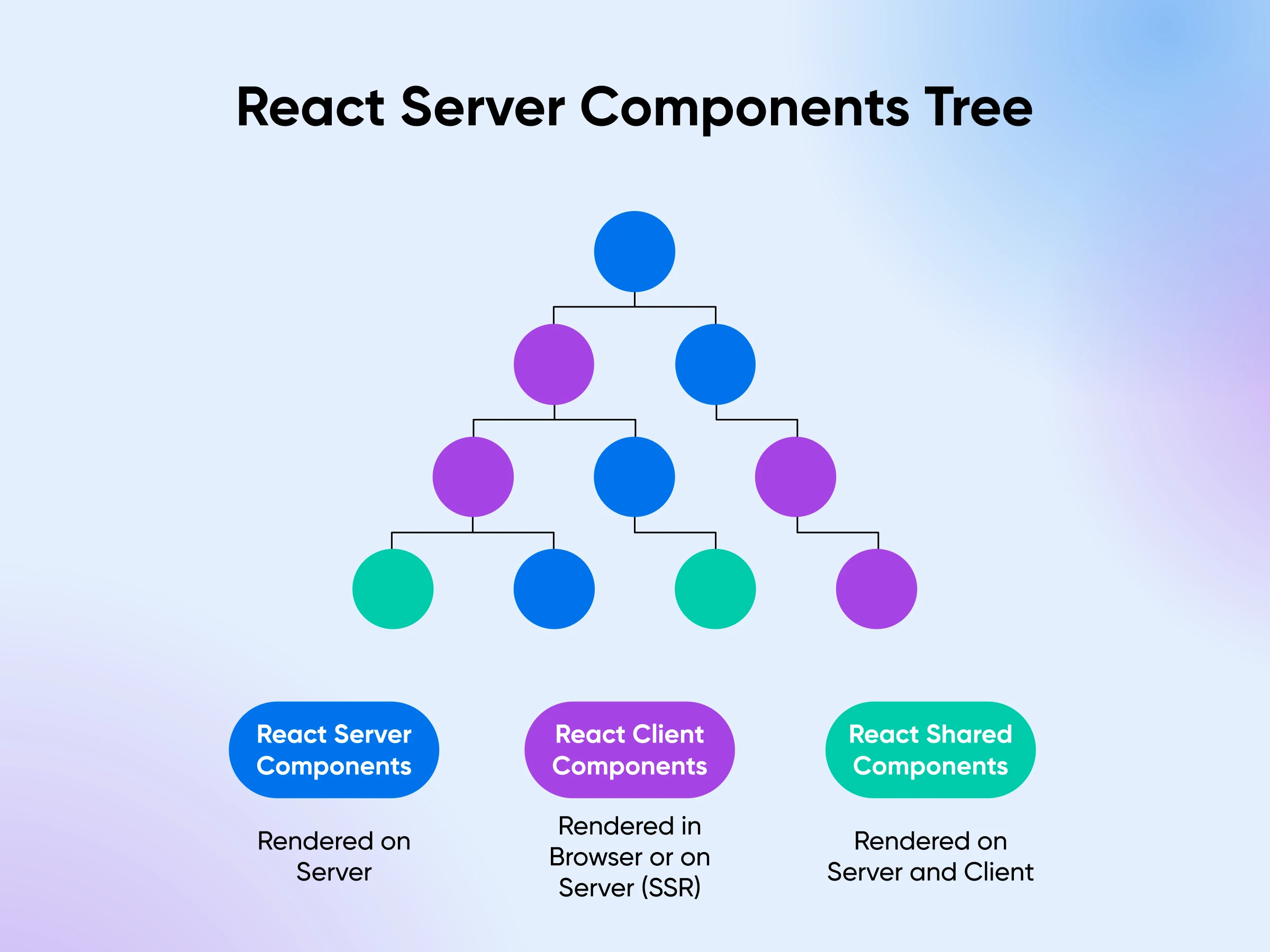
Think of React Server Components as a new way of building React applications. Instead of running in the browser like typical React components, RSCs execute directly on your server.
“I think RSCs are designed to be the “componentization” of the back end, i.e., the back end equivalent of what SPA React did for the front end. In theory, they could largely eliminate the need for things like REST and GraphQL, leading to a much tighter integration between the server and client since a component could traverse the entire stack.” — ExternalBison54 on Reddit
Since RSCs execute directly on the server, they can efficiently access backend resources like databases and APIs without an additional data fetching layer.
API
An Application Programming Interface (API) is a set of functions enabling applications to access data and interact with external components, serving as a courier between client and server.
Read MoreBut why did we need RSCs anyway?
To answer this question, let’s rewind a bit.
Traditional React: Client-Side Rendering (CSR)
React has always been a client-side UI library.
The core idea behind React is to divide your entire design into smaller, independent units we call components. These components can manage their own private data (state) and pass data to each other (props).
Think of these components as JavaScript functions that download and run right in the user’s browser. When someone visits your app, their browser downloads all the component code, and React steps in to render everything:
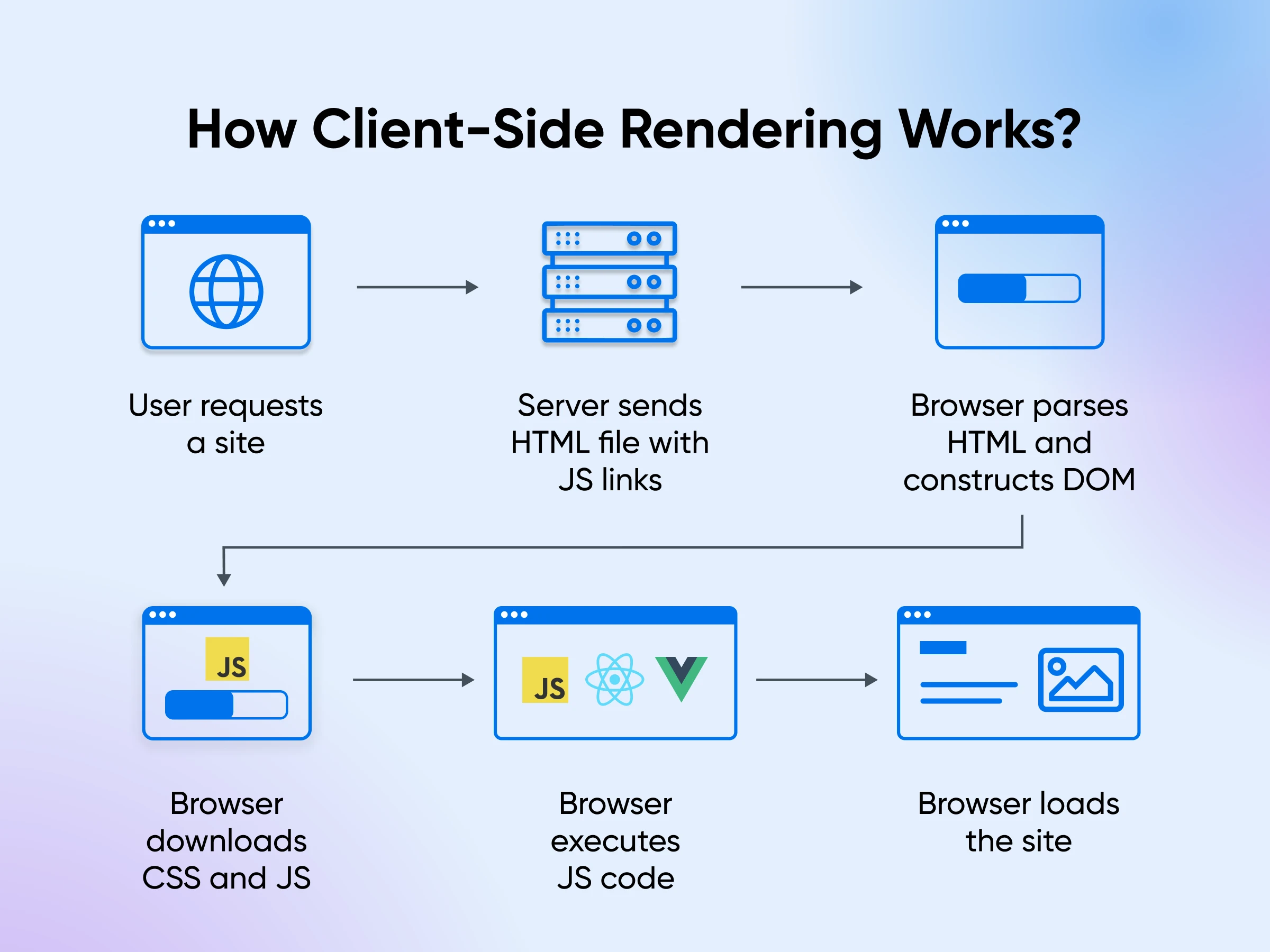
- The browser downloads the HTML, JavaScript, CSS, and other assets.
- React analyzes the HTML, sets up event listeners for user interactions, and retrieves any required data.
- The website transforms into a fully functional React application right before your eyes and everything is done by your browser and computer.
While this process works, it does have some downsides:
- Slow load times: Loading times can be slow, particularly for complex applications with lots of components since now the user has to wait for everything to be downloaded first.
- Bad for search engine optimization (SEO): The initial HTML is often barebones — just enough to download the JavaScript which then renders the rest of the code. This makes it hard for search engines to understand what the page is about.
- Gets slower as apps grow larger: The client-side processing of JavaScript can strain resources, leading to a rougher user experience, especially as you add more functionality.
The Next Iteration: Server-Side Rendering (SSR)
To address the issues caused by client-side rendering, the React community adopted Server-Side Rendering (SSR).
With SSR, the server handles rendering the code to HTML before sending it over.
This complete, rendered HTML is then transferred to your browser/mobile, ready to be viewed — the app doesn’t need to be compiled during runtime like it would without SSR.
Here’s how SSR works:
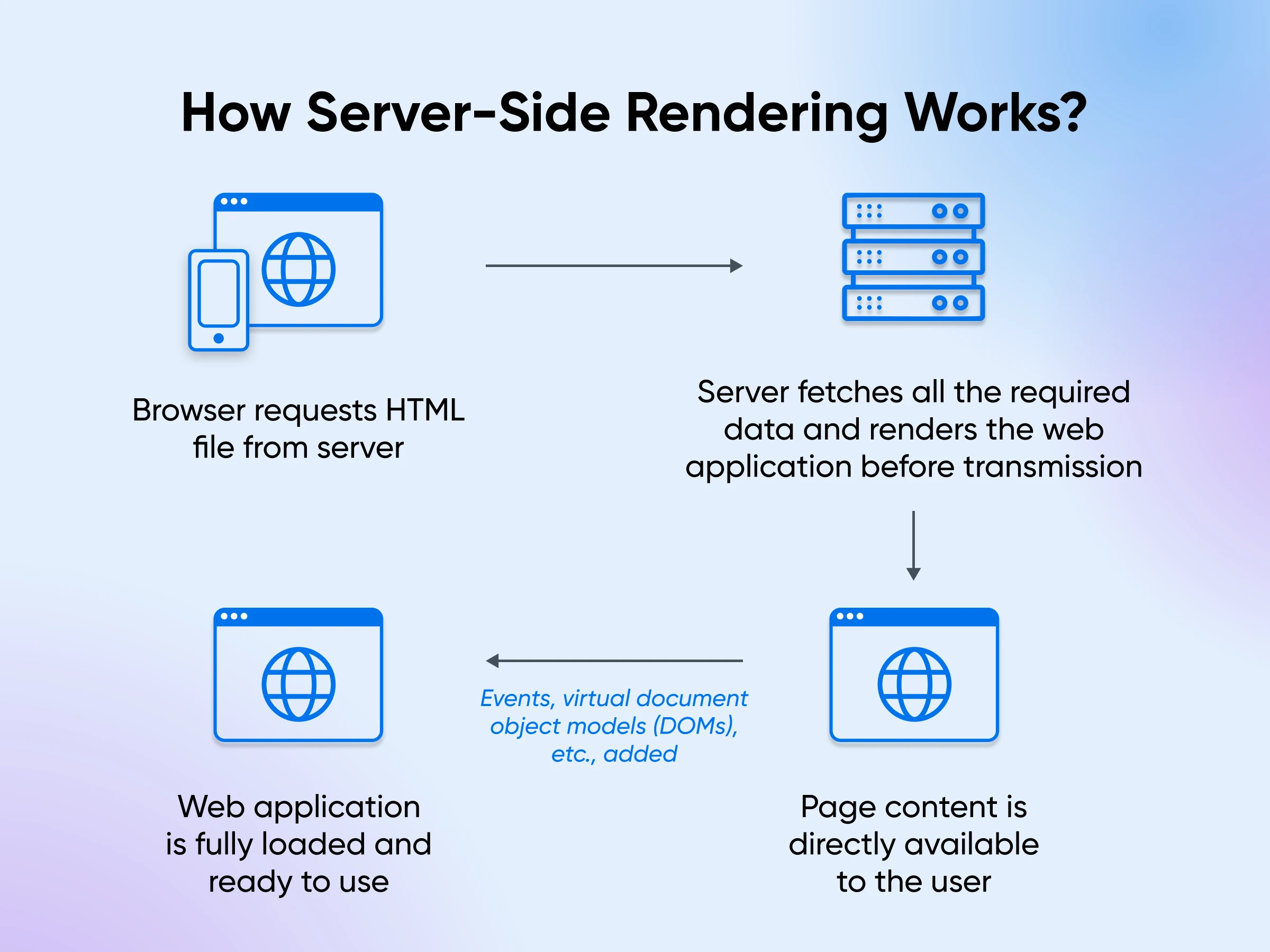
- The server renders the initial HTML for each request.
- The client receives a fully formed HTML structure, allowing for faster initial page loads.
- The client then downloads React and your application code, a process called “hydration,” which makes the page interactive.
The HTML structure rendered on the server has no functionality — yet.
React adds event listeners, sets up internal state management, and adds other functionality to the HTML after it’s been downloaded to your device. This process of adding “life” to the page is known as hydration.
Why does SSR work so well?
- Faster initial load times: Users see the content almost instantly because the browser receives fully formed HTML, eliminating the time required for the JavaScript to load and execute.
- Improved SEO: Search engines easily crawl and index server-rendered HTML. This direct access translates to better search engine optimization for your application.
- Enhanced performance on slower devices: SSR lightens the load on a user’s device. The server shoulders the work, making your application more accessible and performant, even on slower connections.
SSR, however, caused a number of additional problems, calling for an even better solution:
- Slow Time to Interactive (TTI): Server-side rendering and hydration delay the user’s ability to see and interact with the app until the entire process is complete.
- Server load: The server needs to do more work, further slowing down response times for complex applications, especially when there are many users simultaneously.
- Setup complexity: Setting up and maintaining can be more complex, especially for large applications.
Finally, the React Server Components
In December 2020, the React team introduced the “Zero-Bundle-Size React Server Components” or RSCs.
This changed not only how we thought about building React apps but also how React apps work behind the scenes. RSCs solved many problems we had with CSR and SSR.
“With RSCs, React becomes a fully server-side framework and a fully client-side framework, which we’ve never had before. And that allows a much closer integration between the server and client code than was ever possible before.” — ExternalBison54 on Reddit
Let’s now look at the benefits that RSCs bring to the table:
1. Zero Bundle Size
RSCs are rendered entirely on the server, eliminating the need to send JavaScript code to the client. This results in:
- Dramatically smaller JavaScript bundle sizes.
- Faster page loads, particularly on slower networks.
- Improved performance on less powerful devices.
Unlike SSR, where the entire React component tree is sent to the client for hydration, RSCs keep server-only code on the server. This leads to those significantly smaller client-side bundles we talked about, making your applications lighter and more responsive.
2. Direct Backend Access
RSCs can interact directly with databases and file systems without requiring an API layer.
As you can see in the code below, the courses variable is fetched directly from the database, and the UI prints a list of the course.id and course.name from the courses.map:
async function CourseList() {
const db = await connectToDatabase();
const courses = await db.query('SELECT * FROM courses');
return (
<ul>
{courses.map(course => (
<li key={course.id}>{course.name}</li>
))}
</ul>
);
}
This is simpler in contrast to traditional SSR where you’d need to set up separate API routes for fetching individual pieces of data.
3. Automatic Code Splitting
With RSCs, you also get more granular code splitting and better code organization.
React keeps server-only code on the server and ensures that it never gets sent over to the client. The client components are automatically identified and sent to the client for hydration.
And the overall bundle becomes extremely optimized since the client now receives exactly what’s needed for a fully functional app.
On the other hand, SSR needs careful manual code splitting to optimize performance for each additional page.
4. Reduced Waterfall Effect and Streaming Rendering
React Server Components combine streaming rendering and parallel data fetching. This powerful combination significantly reduces the “waterfall effect” often seen in traditional server-side rendering.
Waterfall Effect
The “waterfall effect” slows down web development. Basically, it forces the operations to follow one another as if a waterfall were flowing over a series of rocks.
Each step must wait for the previous one to finish. This “wait” is especially noticeable in data fetching. One API call must be completed before the next one begins, causing page load times to slow.
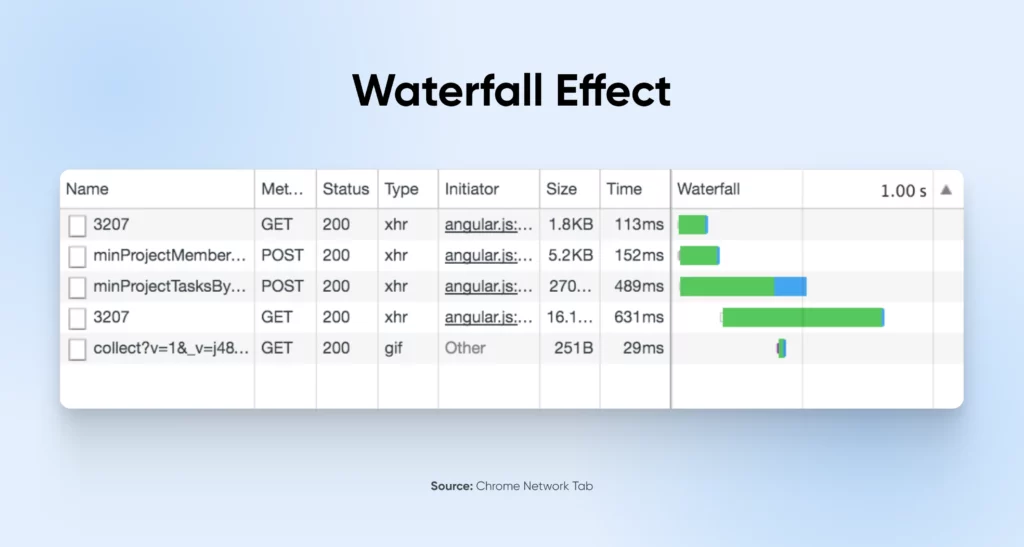
Streaming Rendering
Streaming rendering offers a solution. Instead of waiting for the entire page to render on the server, the server can send pieces of the UI to the client as soon as they’re ready.
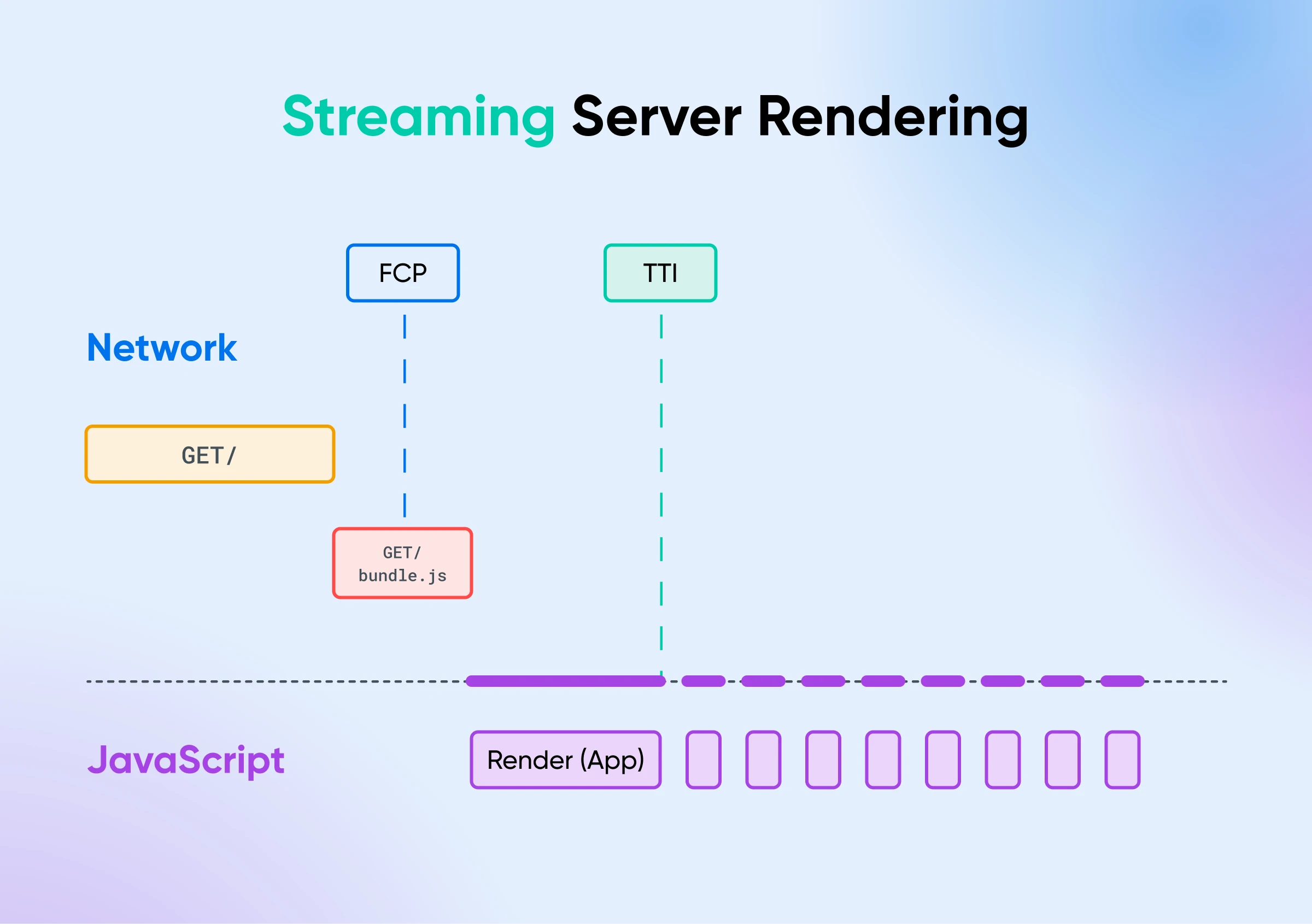
React Server Components make rendering and fetching data much smoother. It creates multiple server components that work in parallel avoiding this waterfall effect.
The server starts sending HTML to the client the moment any piece of the UI is ready.
So, compared to server-side rendering, RSCs:
- Allow each component to fetch its data independently and in parallel.
- The server can stream a component as soon as its data is ready, without waiting for other components to catch up.
- Users see the content loading one after the other, enhancing their perception of performance.
5. Smooth Interaction With Client Components
Now, using RSCs doesn’t necessarily imply that you have to skip using client-side components.
Both components can co-exist and help you create a great overall app experience.
Think of an e-commerce application. With SSR, the entire app needs to be rendered server side.
In RSCs, however, you can select which components to render on the server and which ones to render on the client side.
For instance, you could use server components to fetch product data and render the initial product listing page.
Then, client components can handle user interactions like adding items to a shopping cart or managing product reviews.
Should You Add RSC Implementation on Your Roadmap?
Our verdict? RSCs add a lot of value to React development.
They solve some of the most pressing problems with the SSR and CSR approaches: performance, data fetching, and developer experience. For developers just starting out with coding, this has made life easier.
Now, should you add RSC implementation to your roadmap? We’ll have to go with the dreaded — it depends.
Your app may be working perfectly fine without RSCs. And in this case, adding another layer of abstraction may not do much. However, if you plan to scale, and think RSCs can improve the user experience for your app, try making small changes and scaling from there.
And if you need a powerful server to test RSCs, spin up a DreamHost VPS.
DreamHost offers a fully managed VPS service where you can deploy even your most demanding apps without worrying about maintaining the server.
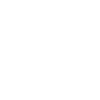
We Know You’ve Got Lots of VPS Options
Here’s how DreamHost’s VPS offering stands apart: 24/7 customer support, an intuitive panel, scalable RAM, unlimited bandwidth, unlimited hosting domains, and SSD storage.
Change Your VPS Plan